Libraries and frameworks greatly simplify and speed up the development process. When it comes to Ethereum development — it is very helpful to use Web3.js. Web3.js is the official library from the Ethereum Foundation. It is a great way to develop a website/client application that interacts with the JSON RPC of the Ethereum blockchain.
In this tutorial, we will learn how to use Web3.js to connect to the Ethereum blockchain using HTTP. We will write code to get the last block number. The most interesting thing about this will be that our code will work for both the front-end (with a build system such as webpack) and the back-end (using NodeJS).
Preinstalled
- NodeJS is installed on your system
- Text editor
- Terminal or command line
Adding web3.js
First you need to get web3.js into your project. This can be done using the following methods:
- npm: npm install web3
- yarn: yarn add web3
- pure js: link the dist/web3.min.js
Get API Key WatchData
To create an API key, you need to do the following steps:On the Dashboard.watchdata.io tab, click Create API key.
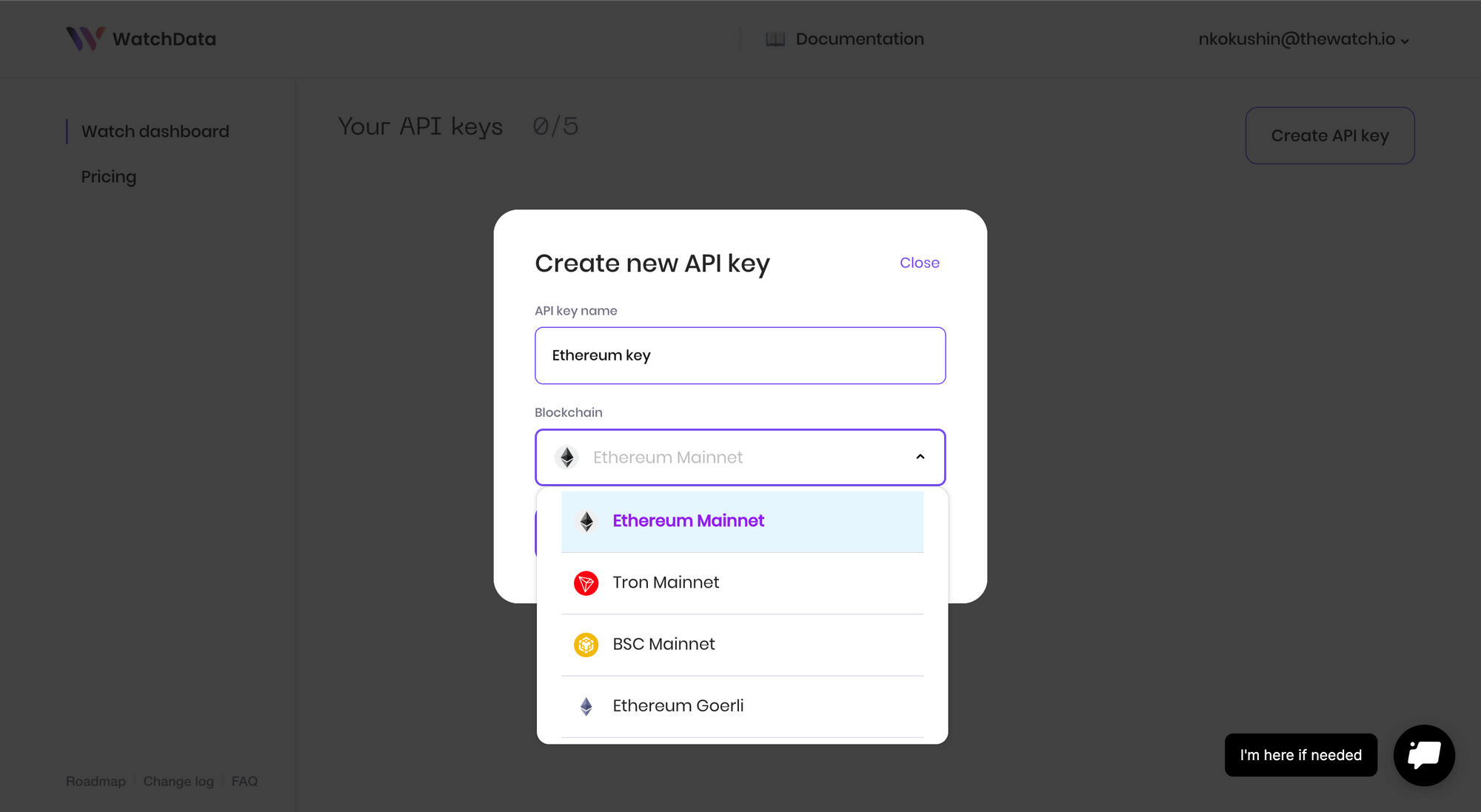
Your API key will be automatically created.
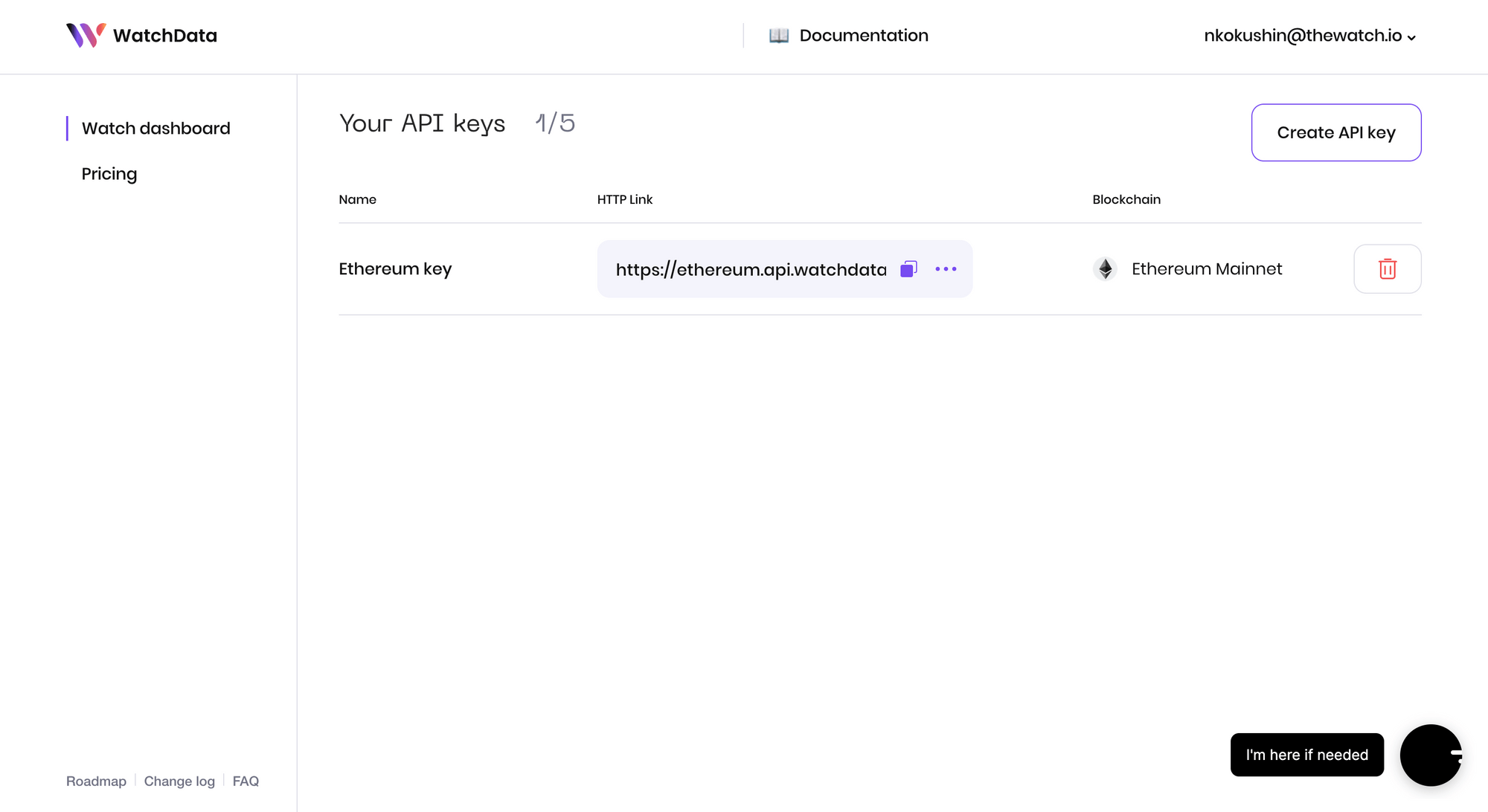
Once you have created the API key, copy it and combine it with the HTTP provider’s endpoint in this format:
For example:
Connecting through Web3
Now let’s create a short script, let’s call it index.js to get the last block number from the blockchain. You can copy/paste this into your code editor:
So go ahead and replace **YOUR_ETHEREUM_NODE_URL** with the http provider from the instructions above.
A brief explanation of the above code: we import the web3 library we installed earlier (line 1), set the URL of our Ethereum host (line 2), create a Web3 HttpProvider instance (line 3) . Finally, we call getBlockNumber for the web3 object and wait for a successful result from the server and then write the result to the console (lines 4–6).
Save this piece of code to an index.js file, which we will run shortly. Run the file with the node command, and you will see the last block number from the Ethereum network:

And so we connected to web3 and got the last block number. Great 😎 You can use Web3.js and WatchData API to develop great applications.